Download images from Google docs and Google Slides
Learn how extract all the embedded images from a Google Document or Google Slides
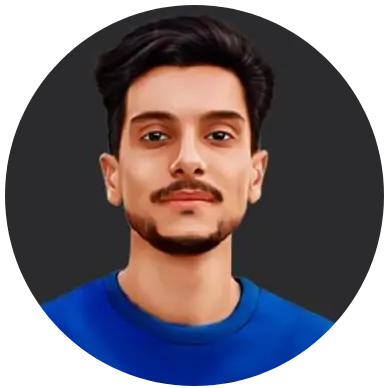
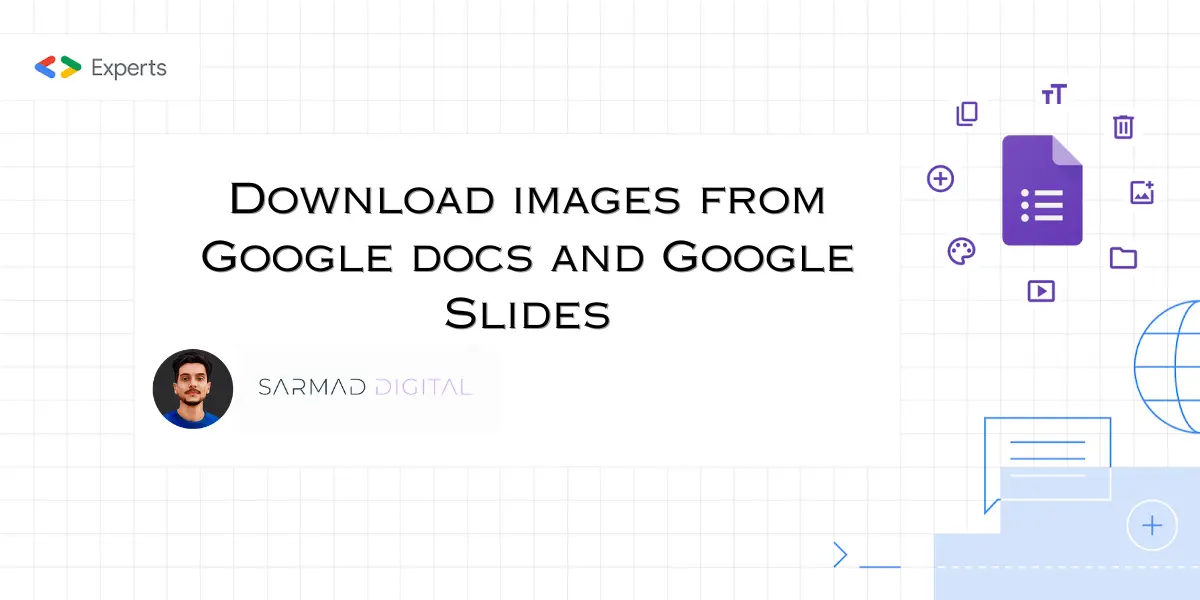
Download images from Google docs and Google Slides
Imagine you’re working with a lengthy Google Document, or a Google Slides presentation, and you need to extract all the embedded images from the text and save them as individual files.
Extract Individual Images
A simple solution to address this issue is as follows: convert your Google Document or Google Slide into a web page. Here’s how you can do it:
Go to the “File” menu. Select the “Share” submenu and then choose “Publish to Web.” It will generate a public web page that contains all the images from your document or slide. You can simply right-click an image on the page and select the “Save Image” option download it to your local disk.
What we have just discussed is a manual process but we can easily automate this with the help of Google Apps Script.
Extract Individual Images
A simple solution to address this issue is as follows: convert your Google Document or Google Slide into a web page. Here’s how you can do it:
Go to the “File” menu. Select the “Share” submenu and then choose “Publish to Web.” It will generate a public web page that contains all the images from your document or slide. You can simply right-click an image on the page and select the “Save Image” option download it to your local disk.
What we have just discussed is a manual process but we can easily automate this with the help of Google Apps Script.
function saveGoogleDocsImages() { // Define the folder name where the extracted images will be saved const folderName = 'Document Images'; // Check if a folder with the specified name already exists const folders = DriveApp.getFoldersByName(folderName); // If the folder exists, use it; otherwise, create a new folder const folder = folders.hasNext() ? folders.next() : DriveApp.createFolder(folderName); // Get all the images in the document's body and loop through each image DocumentApp.getActiveDocument() .getBody() .getImages() .forEach((image, index) => { // Get the image data as a Blob const blob = image.getBlob(); // Extract the file extension from the Blob's content type (e.g., 'jpeg', 'png') const [, fileExtension] = blob.getContentType().split('/'); // Generate a unique file name for each image based on its position in the document const fileName = `Image #${index + 1}.${fileExtension}`; // Set the Blob's name to the generated file name blob.setName(fileName); // Create a new file in the specified folder with the image data folder.createFile(blob); // Log a message indicating that the image has been saved Logger.log(`Saved ${fileName}`); }); }
1234567891011121314151617181920212223242526272829303132333435
Extract all Images from Google Slides
The Apps Script code to download images from a Google Slides presentation is similar. The function iterates over the slides in the presentation and then for each slide, the function iterates over the images in that slide.
function extractImagesFromSlides() { // Define the folder name where the extracted images will be saved const folderName = 'Presentation Images'; // Check if a folder with the specified name already exists const folders = DriveApp.getFoldersByName(folderName); // If the folder exists, use it; otherwise, create a new folder const folder = folders.hasNext() ? folders.next() : DriveApp.createFolder(folderName); // Iterate through each slide in the active presentation SlidesApp.getActivePresentation() .getSlides() .forEach((slide, slideNumber) => { // Retrieve all images on the current slide slide.getImages().forEach((image, index) => { // Get the image data as a Blob const blob = image.getBlob(); // Extract the file extension from the Blob's content type (e.g., 'jpeg', 'png') const fileExtension = blob.getContentType().split('/')[1]; const fileName = `Slide${slideNumber + 1}_Image${index + 1}.${fileExtension}`; // Set the Blob's name to the generated file name blob.setName(fileName); // Create a new file in the specified folder with the image data folder.createFile(blob); Logger.log(`Saved ${fileName}`); }); }); }
123456789101112131415161718192021222324252627282930313233343536