Javascript
Sarmad Gardezi
Convert JPG to WEBP using Google Sheets
Convert JPG to WEBP using Google Sheets using google app script for google sheets
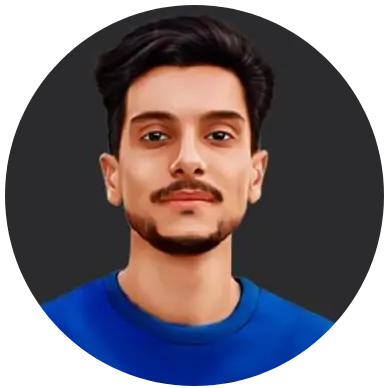
Automate Image Conversion to WebP Format Using Google Sheets and Cloudinary
This script automates the process of converting image URLs in a Google Sheet to WebP format using Cloudinary. It takes image URLs from Column A of the sheet, converts them to WebP, and stores the resulting Cloudinary URLs in Column B.
Steps:
-
Cloudinary Setup:
- Ensure you have a Cloudinary account. Replace with your actual Cloudinary cloud name in the code.
'YOUR_CLOUD_NAME'
1
- Ensure you have a Cloudinary account. Replace
-
Google Sheets Setup:
- Add the original image URLs in Column A of your Google Sheet.
- The script processes these URLs, converts them to , and stores the new links in Column B.
.webp
1
-
Script Breakdown:
- : This function loops through all the URLs in Column A, calls the
convertImagesToWebp()
1function to convert them to WebP format, and stores the new URL in Column B.convertAndGetWebpUrl()
1 - : This function uses Cloudinary's image URL with an explicit conversion to
convertAndGetWebpUrl(imageUrl)
1. It verifies the response before saving the converted URL..webp
1
Code:
// Cloudinary account credentials const cloudName = 'YOUR_CLOUD_NAME'; // Replace with your actual Cloudinary cloud name function convertImagesToWebp() { const sheet = SpreadsheetApp.getActiveSpreadsheet().getActiveSheet(); const lastRow = sheet.getLastRow(); for (let i = 2; i <= lastRow; i++) { const imageUrl = sheet.getRange(i, 1).getValue(); // Get original image URL from Column A if (imageUrl) { // Generate Cloudinary URL with explicit .webp conversion const webpUrl = convertAndGetWebpUrl(imageUrl); // Write the new .webp URL in Column B if (webpUrl) { sheet.getRange(i, 2).setValue(webpUrl); } else { sheet.getRange(i, 2).setValue('Error converting image'); } } } SpreadsheetApp.getUi().alert("All image URLs have been processed."); } function convertAndGetWebpUrl(imageUrl) { try { // Ensure the URL is encoded properly const encodedImageUrl = encodeURIComponent(imageUrl); // Construct the Cloudinary URL for fetching and converting to .webp const cloudinaryBaseUrl = `https://res.cloudinary.com/${cloudName}/image/fetch/f_webp/${encodedImageUrl}`; // Make a request to Cloudinary to check if the URL is valid const response = UrlFetchApp.fetch(cloudinaryBaseUrl, { method: 'GET', muteHttpExceptions: true, }); // Check if Cloudinary returned the expected response if (response.getResponseCode() === 200) { return cloudinaryBaseUrl; // Return the URL if the request was successful } else { Logger.log('Error fetching image from Cloudinary: ' + response.getContentText()); return null; } } catch (error) { Logger.log('Error converting image: ' + error.toString()); return null; } }
1234567891011121314151617181920212223242526272829303132333435363738394041424344454647484950515253
Javascript